(Optional) Create a Before Delete Trigger for Custom Object
For Accounts, Cases, Contacts and Opportunities objects, these triggers have already been provided as part of the S-Drive package.
In this step we'll create a before delete trigger for our custom object. This trigger is used to prevent deletion of the object if it has custom object files in it. This step is an optional step, but we strongly recommend creating this trigger. The following steps will explain the creation of the trigger:
Go to Setup Object Manager tab, find the parent object you’ll use S-Drive with and click on Triggers. Click New.

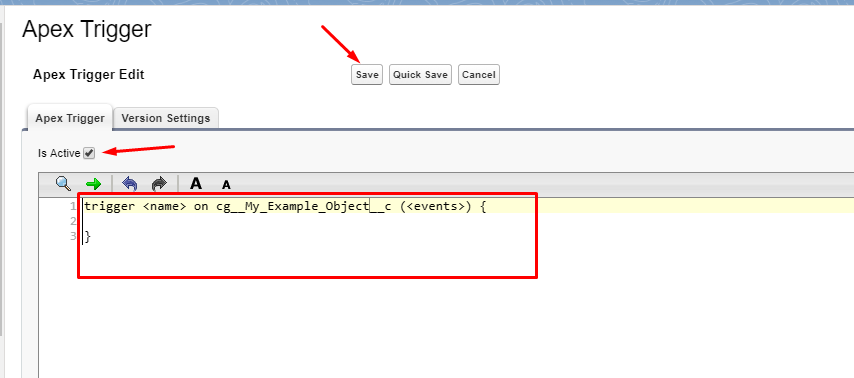
Copy the trigger code in the code block below and paste into your trigger. Make sure you substitute your object for MyObject__c and your S-Drive file object created above for MyObject_File__c in the code below.
trigger MyObjectBeforeDelete on MyObject__c(before delete)
{
List<Id> ids = new List<Id>();
for(MyObject__c obj : Trigger.old)
{
ids.add(obj.Id);
}
Integer tempCount =
[Select count() from MyObject_File__c
where MyObject_File__c.WIP__c = false and
MyOjbect_File__c.Parent__c in :ids];
if(tempCount > 0)
{
Trigger.old[0].addError ('There are S-Drive files attached to this object.' +
' You must delete the files manually before deleting the object!');
}
}
If you have a sandbox environment, and if you must create a test case in order to deploy the trigger to your production organization, below is an example test class for MyObject custom object.
@isTest
private class MyObject_Test {
static testMethod void triggerTest()
{
test.starttest();
MyObject__c obj = new MyObject__c();
obj.Name = 'Test My Ojbect';
insert obj;
MyOjbect_File__c aFile = new MyObject_File__c();
aFile.File_Name__c = 'Test File';
aFile.WIP__c = false;
aFile.Parent__c = obj.Id;
insert aFile;
try
{
delete obj;
system.assert(false); //should never happen
}
catch(Exception e)
{
system.assert(true); //should fail
}
test.stoptest();
}
}